Reusing Design
This guide helps develop concepts for code reuse and de-duplication techniques.
As styles grow, you will gradually find similar class name combinations and the same designs repeated across your codebase.
Abstract styles
When the same composition of styles has the potential to be reused, consider abstracting it.
Avoid abstracting styles of an element prematurely in an attempt to make it look cleaner or reduce code length.
Abstracting into reusable class names
As an example of Master's API for abstracting styles, let's extract this button's styles into a reusable class name.
<button class="inline-flex center-content font:14 font:semibold font:white bg:indigo px:18 h:40 r:4">Submit</button><button class="btn">Submit</button>
import { init, Style } from '@master/css';Style.extend('classes', { btn: 'inline-flex center-content font:14 font:semibold font:white bg:indigo px:18 h:40 r:4'})init()
For the configuration guide, check out Customization Reference.
Appropriate use of this API and reusing components of the same style can make the application's design more consistent, and reduce repeated code.
If abused, excessive abstraction will result in rapid increases in output size. The outputed CSS rules:
.btn, .inline-flex { display: flex }.btn, .font:14 { font-size: 0.875rem }/* ... */
Abstracting into elements
We've provided a syntactic sugar @master/style-element.react, allowing you to quickly build a styled element for reuse.
import React from 'react';import el from '@master/style-element.react';const Button = el.button`inline-flex center-content font:14 font:semibold font:white bg:indigo px:18 h:40 r:4`;export default function App() { return ( <Button className="uppercase">...</Button> <Button className="capitalize">...</Button> );}
rendered as:
<button class="uppercase inline-flex center-content font:14 font:semibold font:white bg:indigo px:18 h:40 r:4">...</button>
<button class="capitalize inline-flex center-content font:14 font:semibold font:white bg:indigo px:18 h:40 r:4">...</button>
We expect to support React, Vue, and any other frameworks.
Abstracting into components
Abstract into reusable components for front-end frameworks such as React, Vue, and Angular, or use a templating language like Ejs, Twig, or Handlebars, etc.
import clsx from 'clsx'; // A tiny utility for constructing className strings conditionally.export default function Button(props) { return ( <button {...props} className={clsx('inline-flex center-content font:14 font:semibold font:white bg:indigo px:18 h:40 r:4', props.className)}> {props.children} </button> )}
Deduplication programming
To reduce code repetition, you can make use of functional programming and a well-designed architecture.
Extract items into loops
Reduce code repetition using loops in a front-end framework like React, Vue, and Angular, or use a template language like Ejs, Twig, or Handlebars, etc.
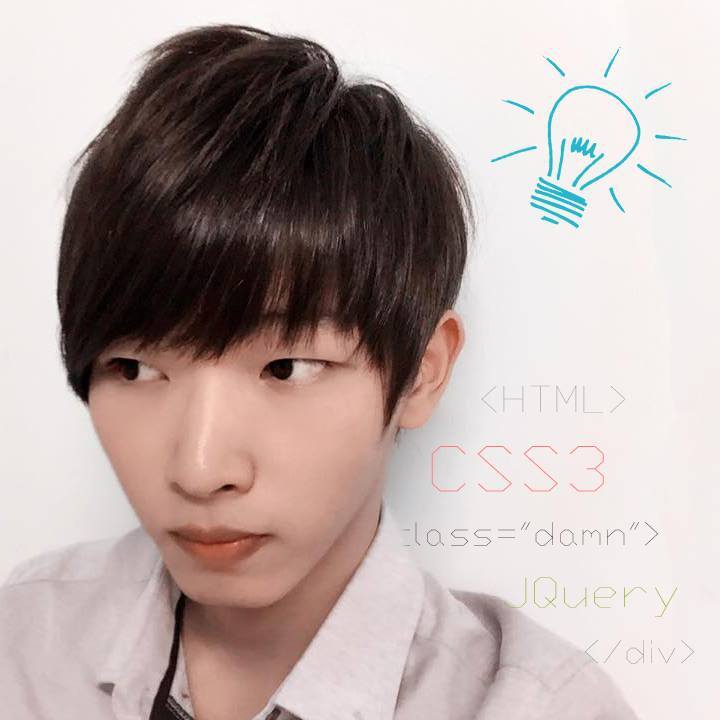
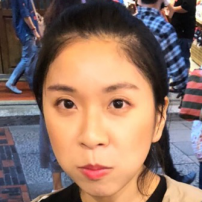
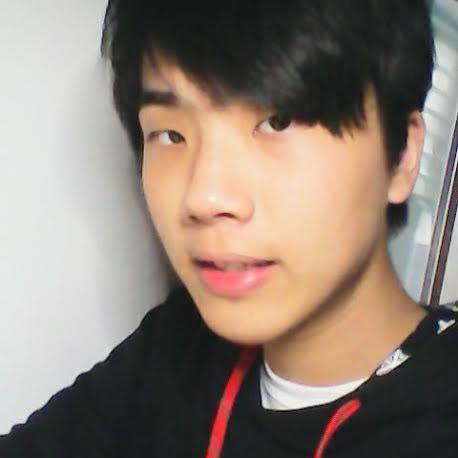
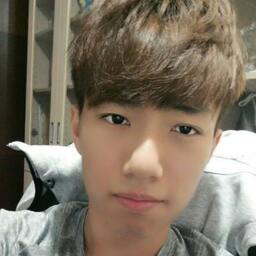
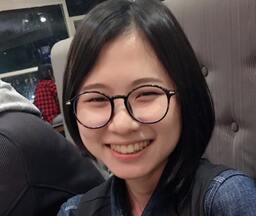
<div className="flex"> <img className="mx:-3 w:48 h:48 round object:cover outline:3|solid|white outline:gray-18@dark" src="/images/users/aron.jpg" alt="aron"> <img className="mx:-3 w:48 h:48 round object:cover outline:3|solid|white outline:gray-18@dark" src="/images/users/joy.jpg" alt="joy"> <img className="mx:-3 w:48 h:48 round object:cover outline:3|solid|white outline:gray-18@dark" src="/images/users/miles.jpg" alt="miles"> <img className="mx:-3 w:48 h:48 round object:cover outline:3|solid|white outline:gray-18@dark" src="/images/users/benseage.jpg" alt="benseage"> <img className="mx:-3 w:48 h:48 round object:cover outline:3|solid|white outline:gray-18@dark" src="/images/users/lola.jpg" alt="lola"> {users.map((user) => <img className="mx:-3 w:48 h:48 round object:cover outline:3|solid|white outline:gray-18@dark" src={user.url} alt={user.name} key={user.name} /> )}</div>